Pattern printing is a great way to improve logic-building skills in Java. It's widely used in coding interviews, competitive programming, and as a fundamental exercise to understand loops and conditional structures.
In this blog, we'll explore some of the most common and interesting pattern problems, with explanations and Java code implementations.
Square Pattern:
A square pattern is one of the simplest patterns, where the same character or number is printed in a grid format. The key idea behind this pattern is using two nested loops:
- The outer loop controls the rows.
- The inner loop controls the columns and prints the character in each row.
This is useful for understanding the basics of nested loops.
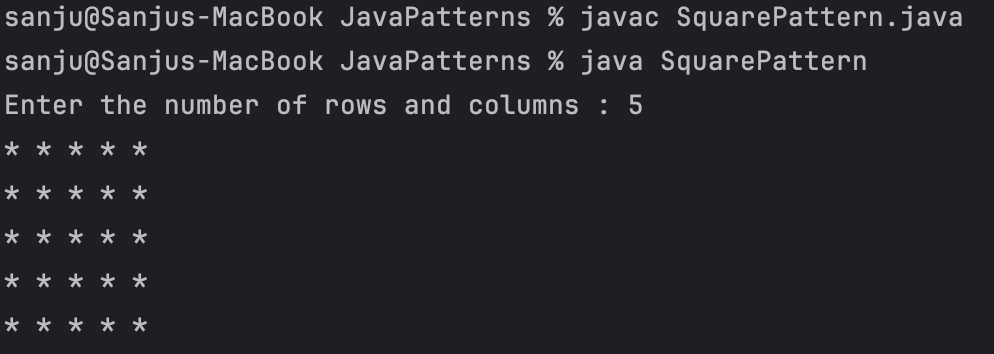
Right-Angled Triangle Pattern:
This pattern builds a right-angled triangle using increasing rows of symbols.
- The number of symbols in each row is equal to the row index.
- The outer loop runs for the total number of rows, while the inner loop prints the increasing count of symbols.
It helps in understanding incremental loop structures.
Inverted Pyramid Pattern:
n this pattern, we start with the maximum number of symbols in the first row, decreasing in each subsequent row.
- The outer loop runs from the maximum row count down to 1.
- The inner loop ensures that each row has fewer symbols than the previous one.
It helps in practicing decremental looping techniques.
Right-Angled Number Pyramid 1:
This pattern is a variation of the right-angled triangle but uses numbers instead of symbols.
- The numbers increase with each row, providing an easy way to understand number-based pattern printing.
It's helpful for working with number sequences in nested loops.
Right-Angled Number Pyramid 2:
This is another numeric pyramid but prints numbers in increasing order, forming a right-angled shape.
- The outer loop controls the number of rows.
- The inner loop ensures that numbers in each row increase progressively.
This enhances logical understanding of number-based patterns.
Inverted Number Pyramid:
This pattern is the inverted form of the numeric pyramid.
- The outer loop runs in decreasing order.
- The inner loop prints numbers based on the row index.
It is an excellent way to practice decrementing loops and number manipulation.