From confusion to confidence this 30-day action plan breaks down exactly what to learn, how to practice, and which tools to master to finally ‘get’ DevOps.
Introduction: The DevOps Learning Curve Is Real (But Fixable)
If you’re trying to learn DevOps and constantly feel like you’re drowning in acronyms, YAML files, and “Hello World” tutorials that go nowhere — you’re not alone.
It’s not just you. DevOps is hard. Not because the tools are inherently impossible, but because most tutorials either:
- assume you already know how everything fits together, or
- dump 27 different tools on you without showing what a real workflow looks like.
You might be jumping between Docker, Kubernetes, Jenkins, Terraform, and GitHub Actions without ever shipping something meaningful. And that’s frustrating.
This guide is different.
I’ve broken down DevOps into bite-sized, no-BS chunks spread over 30 days. You won’t become a guru overnight, but you will understand how to connect the dots, build real projects, and stop Googling “What even is CI/CD?” for the third time this week.
Whether you’re a backend dev trying to go full-stack, a junior engineer who wants to stop fearing the terminal, or just someone who wants to finally deploy something that works, this 30-day plan is for you.
Let’s ditch the hand-wavy diagrams and start building.
Section 2: TL;DR Who This Guide Is For (And What You Should Focus On)
Let’s be real: “DevOps” means different things to different people. Some folks just want to get their app onto a cloud server without breaking everything. Others are staring at Kubernetes and wondering if they’ve accidentally enrolled in wizard school.
Before we go any further, let’s figure out who you are and what parts of DevOps you actually need to learn. This way, you don’t waste your time doing AWS IAM policies when all you wanted was a CI pipeline for your blog.
TL;DR Table Find Your Use Case:
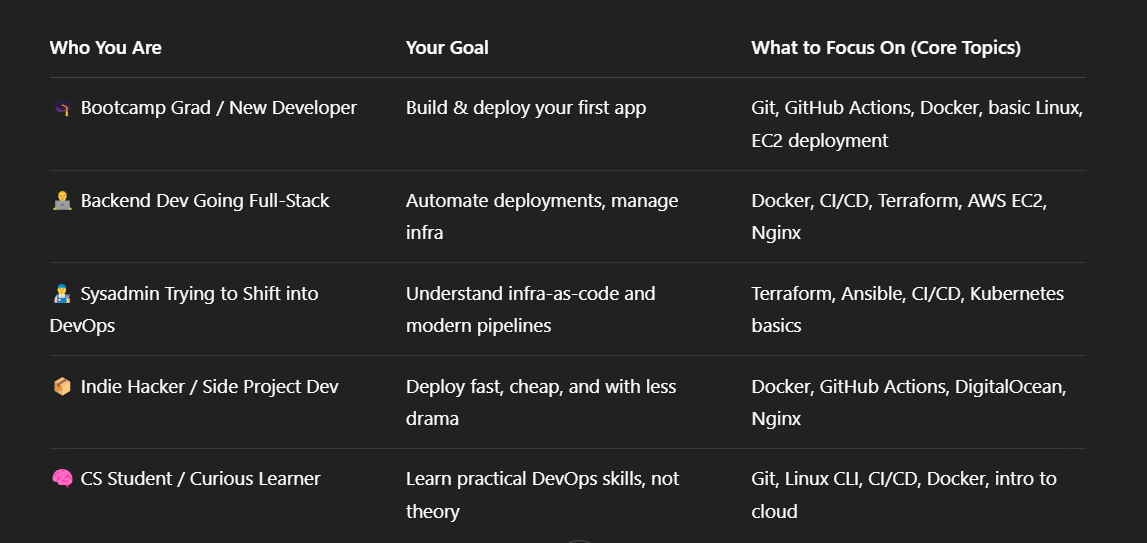
If you’re here just to “learn DevOps” because it’s hot on job boards, do yourself a favor: pick a goal. DevOps is a means to an end, not the end itself.
In the next section, we’ll tear apart the DevOps stack — not with buzzwords, but with real workflows, tool comparisons, and how they connect. You’ll see what you actually need (and what you can ignore for now).
Section 3: The DevOps Stack Breakdown (No Buzzwords, Just Real Stuff)
If you’ve ever stared at a DevOps roadmap and wondered whether you need to learn 15 different tools just to deploy a React app, you’re not alone.
Spoiler: you don’t.
The DevOps world is full of overkill. But when you strip out the hype, most workflows boil down to this one brutal cycle:
Write code → Build → Test → Release → Deploy → Monitor → Repeat
Now let’s break that into actual tools you’ll use, depending on what you’re trying to build and your sanity level.
DevOps Phases & Tool Examples:
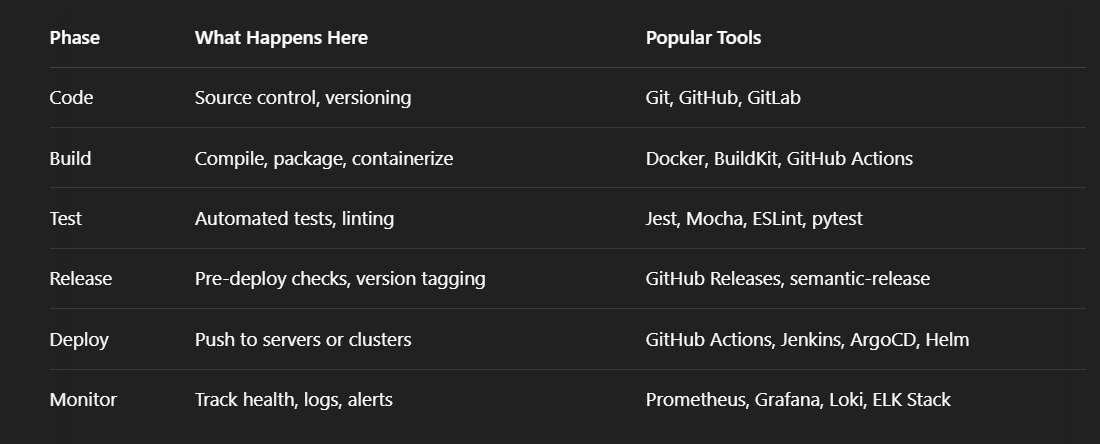
Pro tip: You do not need all these tools. Pick what fits your stack and skill level.
The Stack: Real-World Examples Based on Project Type
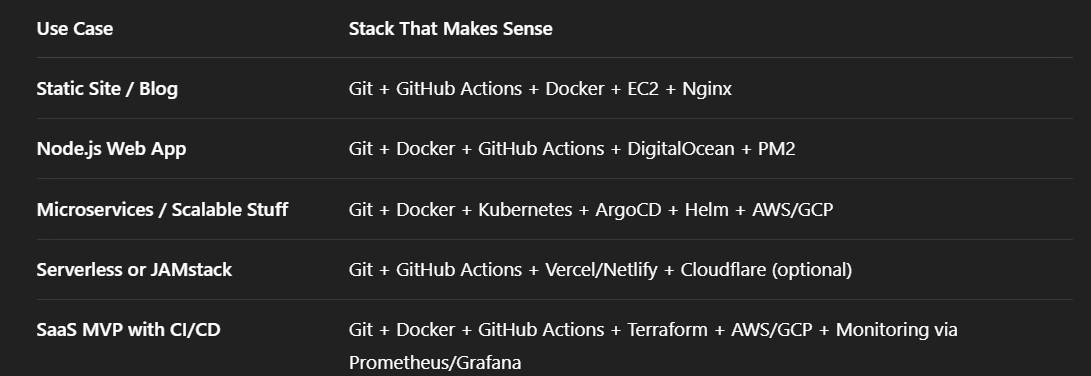
You don’t need to memorize this. You need to build with it. That’s where the 30-day plan comes in.
Section 4: Day 1–5 — Fix the Foundation
Before you try to spin up Kubernetes clusters or write Terraform scripts, take a step back. Most people struggle with DevOps not because of the tools — but because they skipped the basics.
This section is about patching those gaps. Days 1 to 5 are your “let’s stop being afraid of the terminal” phase.
Day 1: Git (For Real This Time)
Yeah, I know — you’ve probably used git add .
and git push origin main
before.
But this time, go deeper:
- Learn what actually happens with staging vs committing
- Practice real branching workflows like
feature/xyz
and pull requests - Merge conflicts? Break stuff on purpose and fix it. It’s the best teacher.
Helpful link: Atlassian’s Git Branching Guide
Day 2: Linux CLI — Your New Best Friend
You will need the command line. It’s unavoidable in DevOps. But good news: most of it is muscle memory.
Practice:
- Navigating with
cd
,ls
,pwd
- File ops like
mv
,cp
,rm
,cat
,touch
- Basic permissions with
chmod
andchown
- Using pipes and redirections:
cat file.txt | grep "ERROR"
is 🔥
Pro tip: Install Ubuntu on WSL (if you’re on Windows) or spin up a cheap VPS to play with real environments.
Day 3: Bash Basics — Automate or Suffer
You don’t have to become a bash wizard, but you should be able to:
- Write a loop
- Use
if
statements - Chain commands
- Write your first basic
.sh
script
Trust me: shell scripting will save your life when you need to deploy at 2 AM.
Day 4: Docker Understand the Hype
Most DevOps jobs mention Docker. Here’s what you really need to know:
- What containers are (hint: not just “lightweight VMs”)
- How to write a Dockerfile from scratch
- How to build and run your own container
- Why your first image is probably bloated (and how to slim it down)
Try this: Dockerize a basic Node.js or Python app. Keep it simple but real.
Official doc: Docker’s Getting Started Guide
Day 5: Docker Volumes, Networks & Best Practices
Now that you’ve got your app in a container, take it a step further:
- Connect containers with Docker networks (ex: app + database)
- Use volumes for persistence (so data doesn’t vanish on restart)
- Learn multi-stage builds to reduce image size
- Clean up old containers/images like a pro (
docker system prune -a
)
This is the day you realize Docker isn’t just about running apps — it’s about portable, repeatable environments.
Section 5: Day 6–10 — CI/CD Demystified (YAML Won’t Kill You, Probably)
Continuous Integration and Continuous Deployment/Delivery — CI/CD — is where DevOps starts to feel like magic and chaos at the same time. Automating your builds and deploys sounds amazing… until your pipeline fails for the 19th time because of an extra space in your YAML file.
Let’s fix that.
Day 6: Understand the CI/CD Flow First
Before you even write a single .yml
file, understand what the pipeline does:
- Detects a change (usually on
push
orpull_request
) - Runs build steps
- Runs tests (if you have them)
- Optionally deploys or pushes artifacts
That’s it. Everything else is just glue around those steps.
Day 7: GitHub Actions — CI That Doesn’t Suck
If you’re using GitHub already, Actions is the fastest way to start:
- Create
.github/workflows/main.yml
- Add a basic workflow that runs on push
- Include a
build
step andecho "It works!"
Then incrementally:
- Add matrix builds (ex: test on Node 16 and 18)
- Set up caching to speed up jobs
- Add secret tokens for deployment (more on this later)
Link to real example: GitHub Actions Node CI
Day 8: GitLab CI / Jenkins / CircleCI — Pick Your Flavor
You might hear people say, “You have to use Jenkins to be a real DevOps engineer.” You don’t.
But here’s when each makes sense:
- GitLab CI if you’re in the GitLab ecosystem (great Docker support)
- CircleCI if you want a polished UI and fast setup
- Jenkins if you love pain (or need advanced customization)
Try one out. Build the same workflow you did in GitHub Actions.
Day 9: Add Tests and Linting to Your Pipeline
CI isn’t just about “did it build?” It’s about quality:
- Run unit tests (use Jest, Mocha, or whatever your language supports)
- Run linters like ESLint or Pylint
- Fail the build if something breaks. Embrace the red ❌ — it’s your friend.
This is where DevOps meets code hygiene. Keep it clean.
Day 10: Deploy Something Automatically
Time to make it real:
- Pick a target: AWS EC2, DigitalOcean, Vercel, Netlify, wherever
- Add a deployment step to your workflow
- Push code → Watch it go live
This feeling? This is why people fall in love with DevOps.
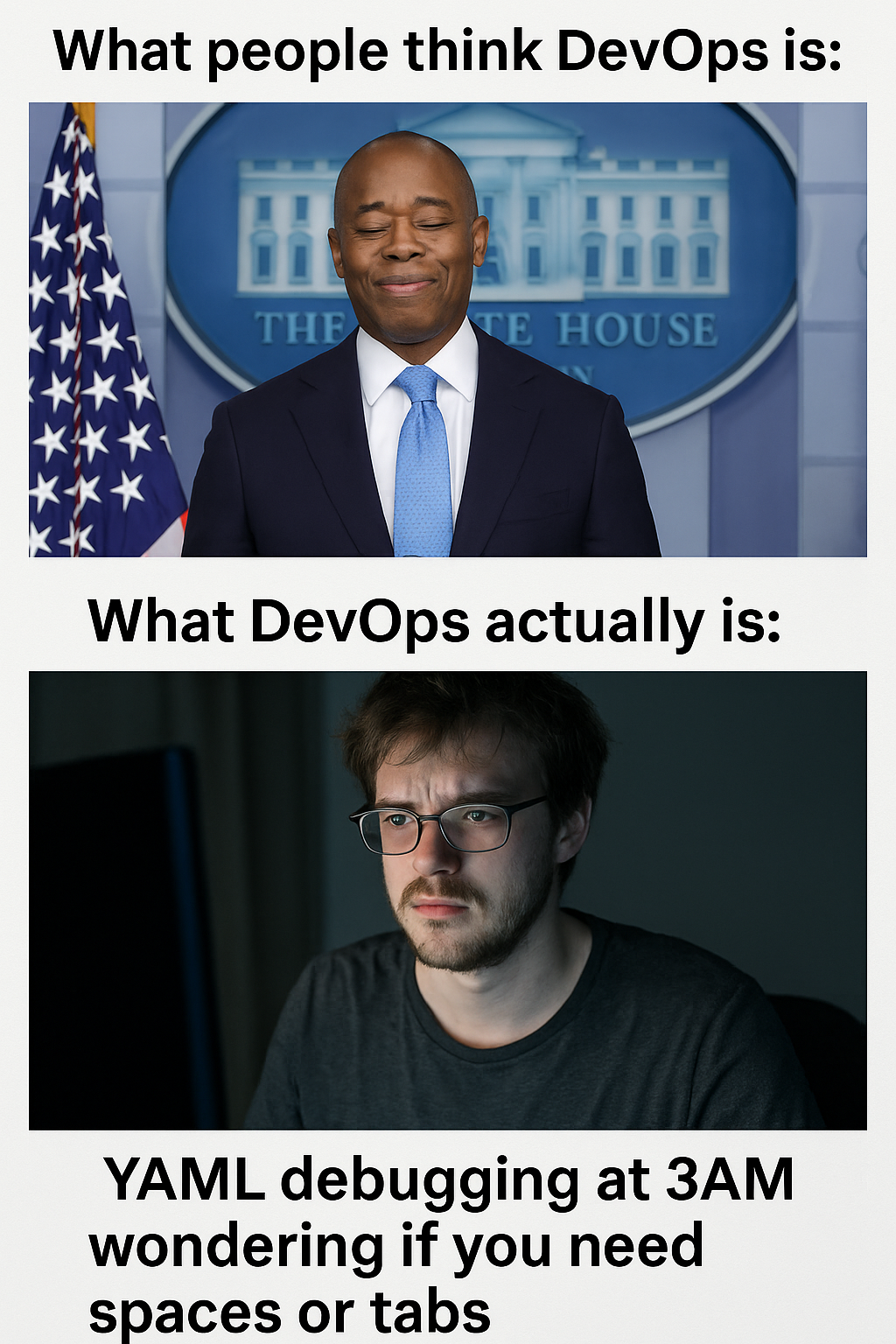
Let me know when you want this meme designed.
Section 7: Day 11–15 Containers & Orchestration (Kubernetes, You Beast)
You’ve now built a pipeline, written YAML, and deployed your app. Feeling powerful? Good. Now let’s see what happens when you want to scale your app across multiple containers, automate rollouts, or simulate an infrastructure apocalypse.
Welcome to the world of orchestration.
Day 11: Docker Deep Dive Beyond Hello World
You’ve already built and run a container — but now it’s time to:
- Use multi-stage builds to optimize your images
- Set up bind mounts and volumes for persistent data
- Create a custom Docker network to connect your app + DB
- Learn about health checks and why they matter in orchestration
Also, get used to tagging your images properly. :latest
is the silent killer.
Day 12: Intro to Kubernetes Yes, It’s Intimidating (At First)
Kubernetes isn’t just Docker on steroids. It’s a whole orchestration engine that manages containers at scale. But we’ll take it one controlled explosion at a time.
Start with:
- kubectl and what it actually does
- Creating your first Pod, Service, and Deployment
- Understanding why YAML is back (you thought you were done, huh?)
- Using Minikube or Kind to run K8s locally
Helpful link: Kubernetes Basics Interactive Tutorial
Day 13: Deploy a Real App to K8s
Take the app you containerized earlier and:
- Write a simple Kubernetes deployment YAML
- Expose it via a NodePort or LoadBalancer (locally for now)
- Scale it up with
kubectl scale deployment
- Break it and fix it. Intentionally. It’s how you learn.
Bonus points if you use a database with persistent storage.
Day 14: Helm Kubernetes, But With Training Wheels
Tired of copy-pasting the same YAML over and over? Say hello to Helm, the package manager for Kubernetes.
With Helm, you can:
- Use pre-built “charts” to deploy apps like MongoDB or Redis in seconds
- Customize deployments with
values.yaml
- Roll back changes without rewriting 50 lines of YAML
Think of it as the npm install
of the K8s world.
Day 15: ArgoCD GitOps for the Win
If you like the idea of using Git as the single source of truth, try ArgoCD. It’s a declarative GitOps tool for Kubernetes.
What it gives you:
- Automated sync between Git and your cluster
- Visual UI to track deployments and changes
- Clean rollback options when stuff breaks (and it will)
Is it necessary on Day 15? No. But it’s worth knowing it exists. You’ll run into it sooner or later in production setups.
You’ve officially gone from “I can’t even Docker” to running a containerized app on Kubernetes. Not bad for two weeks.
Section 8: Day 16–20 — Infrastructure as Code (ClickOps Is Cancelled)
If you’ve ever spent 30 minutes setting up an EC2 instance and then realized you forgot to open port 80, you already understand the pain that Infrastructure as Code (IaC) solves.
IaC is about turning your cloud infrastructure into version-controlled, repeatable code. You build, destroy, and rebuild infra without clicking anything.
Let’s get into it.
Day 16: What the Heck Is Infrastructure as Code?
In plain English:
- You write files (usually in HCL, YAML, or TypeScript)
- Those files describe your infrastructure (servers, DBs, VPCs, etc.)
- You apply them with a tool, and the infra just appears
Benefits:
- No more “works on my AWS account” situations
- Instant rollback of infra changes
- You can finally sleep at night knowing everything is in Git
Day 17: Terraform — Your First Real IaC Tool
Terraform is the king of IaC right now, and for good reason:
- Cloud-agnostic (works with AWS, GCP, Azure, etc.)
- Declarative syntax (describe what you want, not how to get it)
- Large community, tons of modules
Start small:
- Install Terraform
- Write a file to create an AWS EC2 instance
- Run
terraform init
,plan
, andapply
- Destroy it with
terraform destroy
and laugh at your power
Official guide: Terraform Getting Started
Day 18: Variables, Outputs, and State Management
Terraform gets real very quickly:
- Use
variables.tf
to avoid hardcoding - Use
outputs.tf
to return important info like public IPs - Understand state files — they track what Terraform manages
Pro tip: Never commit .tfstate
to Git. Store it in S3 or use Terraform Cloud.
Day 19: Terraform vs Pulumi vs CloudFormation (Pick Your Poison)
Let’s keep this human and honest:
- Terraform = solid, boring, works well
- Pulumi = for devs who hate YAML and love writing infra in TypeScript/Python
- CloudFormation = AWS’s native option, great for locking yourself into their ecosystem
Use Terraform unless you have a very specific reason not to.
Day 20: Build a Full Infra Setup
Let’s put it all together:
- Create a VPC, subnet, and EC2 instance
- Open security group ports (80, 443, etc.)
- Add a Route53 DNS record
- Deploy your Dockerized app to the instance
Bonus: Hook this into your CI/CD workflow from earlier so it auto-deploys.
You now control the cloud with code. You’ve gone from “I need to Google how to open ports” to writing infra that self-deploys, self-documents, and scales.
Section 9: Day 21–25 — Monitoring, Logging, and Not Losing Your Mind
Congrats! Your app is live.
But now comes the moment every DevOps engineer dreads: something goes wrong… and nobody knows why. Your EC2 instance is humming along quietly or is it?
Here’s where observability steps in. Monitoring and logging aren’t just fancy dashboards. They’re your early-warning system before your users tell you something’s broken on Twitter.
Day 21: Start With the Basics — Metrics, Logs, and Alerts
Here’s what you really need:
- Metrics = numbers you care about (CPU, memory, response times)
- Logs = the diary of your app (errors, warnings, debug info)
- Alerts = when things hit the fan and you need to wake up
Focus on signal, not noise. Too many alerts will train you to ignore them.
Day 22: Monitoring With Prometheus + Grafana
This combo is beloved for a reason:
- Prometheus scrapes metrics from your apps and systems
- Grafana makes those metrics look awesome (and readable)
What to do:
- Run both using Docker Compose
- Export basic metrics from your app (via
/metrics
endpoint) - Build a dashboard to visualize CPU usage, uptime, etc.
You’ll feel like Tony Stark watching your app in real time.
Helpful link: Prometheus + Grafana setup guide
Day 23: Logging With the ELK Stack (Elasticsearch, Logstash, Kibana)
The ELK stack gives you:
- Centralized logs from all your services
- Searchable history to trace issues
- Dashboards to visualize log volume, error spikes, and more
Start with:
- Filebeat or Fluentd to ship logs from your app
- Elasticsearch to store them
- Kibana to visualize it all
Warning: This stack is resource-heavy. Start small or use a managed service like Logz.io if you’re just experimenting.
Day 24: Set Up Alerts That Don’t Annoy You
Whether you’re using Prometheus Alertmanager, Grafana alerts, or AWS CloudWatch:
- Only alert on actionable events
- Use thresholds and time windows to avoid flapping
- Route alerts to Slack or email (PagerDuty if you’re fancy)
Don’t be that person who gets 74 alerts and ignores them all.
Day 25: Incident Response Don’t Panic, Plan
Now simulate a failure:
- Kill your app pod/container
- Watch what breaks (or doesn’t)
- Create a basic incident checklist: logs to check, metrics to monitor, restart strategy
Bonus tip: Use UptimeRobot or StatusCake for external uptime checks.
At this point, your app is not only running it’s surviving. You know when it breaks, you know why it breaks, and you (probably) know how to fix it.
Section 10: Day 26–29 — Real-World Projects to Prove You Can Actually Do This Stuff
By now, you’ve collected a toolbox full of shiny DevOps skills. But here’s the thing skills don’t stick unless you build with them. So for the next 4 days, we’re turning theory into muscle memory.
These projects aren’t perfect. That’s the point. You’ll troubleshoot, Google errors, and yell at YAML. But when you’re done, you’ll have something real you can deploy, showcase, and expand.
Project 1: Static Site with CI/CD + Docker + EC2 + Nginx
Goal: Deploy a portfolio, blog, or any static site using real DevOps practices.
What you’ll do:
- Write a GitHub Actions pipeline that builds and pushes a Docker image of your static site
- Use Terraform to spin up an EC2 instance with security groups and SSH access
- Install Docker + Nginx on the instance (with a bash script or Ansible)
- Pull the latest image and serve it via Nginx
Bonus: Set up a domain with Route53 and use Let’s Encrypt for HTTPS.
Project 2: Fullstack App on Kubernetes (Mongo + Express + React)
Goal: Deploy a microservice-based app on a local or cloud Kubernetes cluster.
What you’ll do:
- Containerize all three services
- Write Kubernetes manifests (Deployment + Service) for each one
- Use a K8s Ingress controller to route traffic
- Use Helm to manage configs and deployment
- Optionally, expose a metrics endpoint and view it in Prometheus/Grafana
Bonus: Break one of the services and fix it using logs and kubectl.
Project 3: GitOps with ArgoCD
Goal: Go pro with GitOps and stop manually kubectl-ing everything.
What you’ll do:
- Install ArgoCD on your cluster
- Set up a Git repo with your K8s manifests or Helm charts
- Point ArgoCD at that repo and watch it auto-sync
- Make a change in Git → it auto-deploys to your cluster
Bonus: Roll back a broken deployment using ArgoCD’s UI. No terminal needed.
Project 4 (Optional): CI/CD + Terraform + Monitoring End-to-End Infra
Goal: Go full-stack DevOps. Cloud-native. Infrastructure as code. Monitoring. The works.
What you’ll do:
- Use Terraform to create AWS infrastructure (VPC, EC2, RDS)
- Deploy a containerized app via GitHub Actions pipeline
- Set up Prometheus + Grafana for metrics
- Add alerts to catch high CPU or downtime
- Log everything with the ELK stack
By the end, you’ll basically have a mini SRE playground.
These aren’t just “portfolio projects.” These are battle-tested labs that mirror real DevOps workflows. Share them, blog them, iterate on them. They’ll help you stand out in job hunts and level up faster than theory ever could.
Now, on Day 30, we’ll wrap things up: what comes after the 30 days, where to go next, and how to not fall into the imposter syndrome trap.
Section 11: Day 30 What Now? (You’re Not a Noob Anymore)
So here you are — 30 days later. You’ve wrangled Docker, survived YAML, tamed Terraform, dipped your toes into Kubernetes, and set up enough logging to rival NASA. You’ve probably broken things. Fixed things. Googled errors with more desperation than you’d like to admit.
But here’s the good news: you now understand DevOps in practice — not just in theory.
What You’ve Accomplished in 30 Days
- You’re writing infrastructure as code.
- You’ve automated deployments.
- You understand monitoring, logging, and alerting.
- You can explain CI/CD without sweating.
- You’ve deployed real-world apps, not just toy demos.
That’s more than what most “DevOps engineers” on paper can actually do.
You didn’t just memorize tools — you used them. And that makes all the difference.
What Comes Next?
Now that you’ve got momentum, keep building. Here are some next-level paths depending on what lights you up:
Want to go deep into cloud?
→ Start preparing for certifications like AWS Certified DevOps Engineer
Want to work on large-scale infra?
→ Explore service meshes like Istio, Kubernetes Operators, and distributed tracing (OpenTelemetry)
Want to freelance or consult?
→ Master cost optimization, security best practices, and multi-cloud deployments
Want to work at a startup?
→ Get great at shipping fast, managing CI/CD pipelines, and debugging broken infra with minimal tools
Whatever you do, don’t stop building.
Avoid the Trap: Imposter Syndrome Is a Liar
You will feel like you still don’t know enough. That’s normal. DevOps isn’t a finish line it’s a loop (just like your CI/CD pipeline).
What matters is:
- You know how to troubleshoot
- You know how to ask better questions
- You’ve done the work
That’s 90% of being great at DevOps.
Section 12: Helpful Resources (No Sponsored Trash, Promise)
Here’s a list of legit books, tutorials, tools, docs, and communities to take your DevOps skills beyond Day 30.
Books Worth Reading
- The Phoenix Project — A legendary intro to DevOps, told like a novel. Read it even if you hate books.
- The DevOps Handbook — More tactical and real-world. Perfect follow-up to Phoenix Project.
- Kubernetes Up & Running — The best book to go from “wtf is a pod” to deploying apps on K8s.
GitHub Repos That Slap
- Awesome DevOps A massive curated list of DevOps tools
- Awesome CI/CD Everything from Jenkins to GitOps
- DevOps Exercises Interview prep + practical questions
- Kubernetes the Hard Way Learn K8s like a boss
Communities Where DevOps Nerds Hang Out
- r/devops — Unfiltered, real discussions (and memes)
- Stack Overflow — Good for answers, bad for your ego
Final Advice
- Don’t chase tools. Chase understanding.
- Don’t panic when things break. That’s the job.
- Document everything. Future-you will thank past-you.
- Stay curious. This industry evolves every 6 months.
That’s a Wrap!
If you made it this far: congrats. You didn’t just survive 30 days of DevOps — you leveled up with actual skills.
This isn’t the end. It’s your starting point.