No fluff, just simple explanations, real commands, and tips that make Docker easy to learn and use.
Introduction
Docker is everywhere. Whether you’re building websites, mobile apps, or backend services, chances are someone on your team is using Docker or wants to use it but is too scared to ask.
You’ve probably seen tutorials throwing around terms like “images,” “containers,” and “volumes,” and thought:
“Is this DevOps wizardry or some kind of Linux black magic?”
Don’t worry — this guide breaks Docker down in plain English.
We’ll go from:
- running your first container
- to writing your own Dockerfiles
- to using Docker Compose
- and even touching advanced tricks pros use in real projects.
And yes, there’s a meme too.
Whether you’re a total newbie or someone who’s kinda used Docker but never felt confident, this is for you.
Let’s start your Docker journey one container at a time.
Section 2: Docker 101 Getting Your Hands Dirty
So, what is Docker?
In simple words:
Docker lets you package your app with everything it needs — code, libraries, system tools — into a single, portable unit called a container. That container can run anywhere: your laptop, your teammate’s laptop, a server, or the cloud and it’ll work exactly the same.
Think of it like:
🎮 A video game emulator that runs the same game the same way no matter what computer it’s on.
Why not just install stuff normally?
Because normal setups are messy. One dev has Node.js 16, another has 18, and suddenly something breaks. Docker says, “Let’s put your app in its own bubble so it doesn’t care what’s outside.”
Containers vs. Virtual Machines
FeatureDocker (Containers)Virtual MachinesSpeedSuper fastSlowerSizeLightweight (MBs)Heavy (GBs)Boot timeSecondsMinutesUses system RAMLessMoreBest forDev & microservicesFull OS testing
So yeah, Docker is like the lightweight ninja. VMs are the big tanks.
Installing Docker (Quick Links)
Pick your system and follow the official guide:
- Windows
- Mac
- Linux
Once installed, open a terminal and try this magic:
docker run hello-world
If all goes well, you’ll see a cheerful message from Docker.
Congrats, you’ve just run your first container 🎉
Basic Commands to Remember
CommandWhat it Doesdocker ps
See running containersdocker stop
Stop a running containerdocker images
List your downloaded imagesdocker rmi
Remove an imagedocker exec -it
Open terminal inside a running container
You don’t need to memorize these. You’ll pick them up fast just by using them a few times.
Next up: We go deeper into images vs containers, and you’ll learn to write your own Dockerfile.
And yeah that’s where things start to feel powerful.
Section 3: Images & Containers — The Real MVPs
You hear people say “images” and “containers” like they’re interchangeable. But they’re not the same — and understanding the difference is what takes you from beginner to “I know what I’m doing.”
What’s a Docker Image?
A Docker image is like a recipe or blueprint.
It’s a read-only file that contains everything your app needs — code, runtime, dependencies, environment variables, etc.
You can’t run it directly just like you can’t eat a recipe.
But once you “cook” it into a container, it’s game on.
What’s a Docker Container?
A container is a running instance of an image.
It’s your app, actually alive and doing stuff.
So:
- Image = the instructions
- Container = the app built and running from those instructions
Real-World Analogy:
Image = the game disc
Container = you playing the game on your console
You can make as many containers as you want from one image, just like you can play the same game on multiple consoles.
Creating Your Own Docker Image
Let’s say you have a simple Node.js app. Here’s what a basic Dockerfile
might look like:
# Use an existing image as base
FROM node:18
# Set working directory
WORKDIR /app
# Copy files
COPY package.json ./
RUN npm install
COPY . .
# Expose a port
EXPOSE 3000
# Start the app
CMD ["npm", "start"]
Save this as Dockerfile
in your project folder. Then run:
docker build -t my-node-app .
Boom. You just built a custom Docker image.
Now spin it up:
docker run -p 3000:3000 my-node-app
If everything works, your app is now running inside a container 🚀
Size Does Matter — Image Layers
Docker images are built in layers. Every line in your Dockerfile adds a new layer. This helps with caching — so Docker doesn’t rebuild everything from scratch every time.
Example:
- You change just one file? Only the final layers get rebuilt.
- You change the base image? Everything rebuilds.
That’s why ordering in your Dockerfile matters. Place frequently changing lines below the ones that rarely change.
Best Practices to Level Up
- Use
.dockerignore
to avoid copyingnode_modules
,.git
, or large files - Always tag your images (e.g.,
my-app:dev
,my-app:v1
) - Don’t run containers as root (we’ll get to that later)
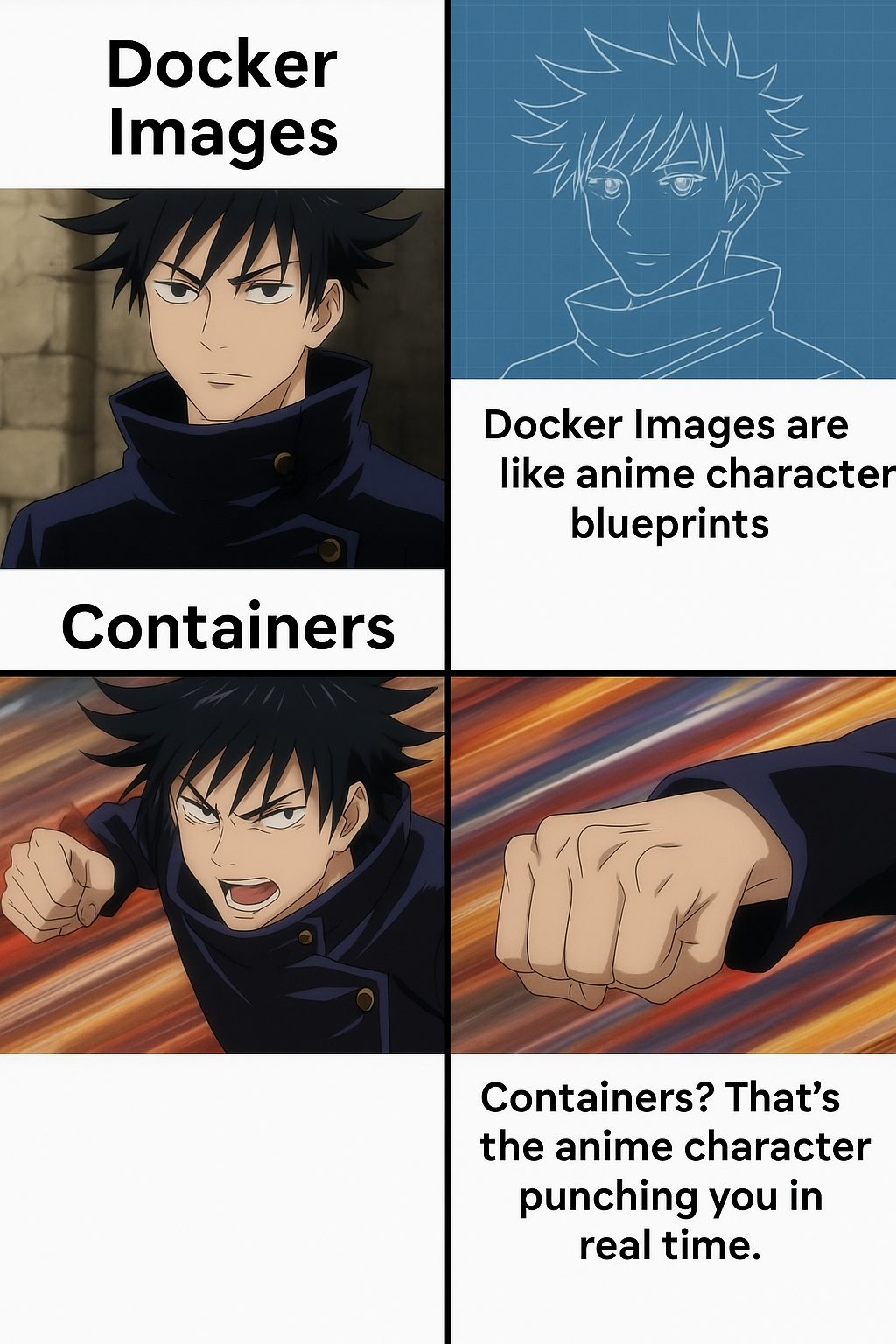
Section 4: Volumes, Networks & Compose Getting Serious
You’ve built an image and run a container — nice.
Now let’s talk about how to store data, connect services, and avoid typing 10 commands every time.
Volumes Save Your Data (So It Doesn’t Vanish)
By default, when a container dies, all its data dies with it.
You restart your app and boom, your database is empty again. Not fun.
Docker volumes fix that.
They let you save data outside the container, so even if the container is removed, your data survives.
Basic volume example:
docker run -v mydata:/app/data my-app
Or to map to a real folder on your machine:
docker run -v $(pwd)/data:/app/data my-app
Now your app can read/write to /app/data
, and it’ll stick around.
Networks Let Containers Talk to Each Other
Let’s say you’ve got a Node.js app and a MongoDB container.
They need to talk — but how?
Docker networks = private LAN party for your containers.
docker network create my-network
Then run both containers with the same network
docker run -d --network my-network --name mongodb mongo
docker run -d --network my-network my-node-app
Now the Node app can connect to mongodb:27017
.
No IP address magic needed.
Docker Compose The Real Time Saver
Tired of typing long commands to run multiple services?
Docker Compose lets you define everything in one file.
Here’s a simple docker-compose.yml
for a Node + Mongo stack:
version: '3'
services:
app:
build: .
ports:
- "3000:3000"
depends_on:
- mongo
networks:
- devnet
mongo:
image: mongo
volumes:
- mongo-data:/data/db
networks:
- devnet
volumes:
mongo-data:
networks:
devnet:
Just run:
docker-compose up
And boom — it spins up your app and the database.
No more command line spaghetti 🍝
Real Use Case
You’re building a full-stack app. With Compose:
- You spin up the backend
- Launch the frontend
- Boot the database
- All in one line
You can even scale services (docker-compose up --scale app=3
) or link to reverse proxies like Nginx.
Pro Tips
- Use named volumes for persistent DB data
- Use
.env
files to keep secrets and ports tidy - Keep Docker Compose files version-controlled
Section 5: Dev Workflows with Docker — Level Up Time
You’ve got Docker basics down. Now let’s use it like real devs do — to build, test, debug, and run apps with less pain and more power.
Here’s how pros use Docker in their day-to-day work.
Dockerizing Real Apps
Let’s look at how to Dockerize apps in the most popular dev languages.
🔵 Node.js
Basic structure:
pgsql
📁 my-app
├── Dockerfile
├── package.json
└── index.js
Dockerfile:
Dockerfile
FROM node:18
WORKDIR /app
COPY package.json ./
RUN npm install
COPY . .
EXPOSE 3000
CMD ["node", "index.js"]
Build and run:
docker build -t my-node-app .
docker run -p 3000:3000 my-node-app
Python
Dockerfile:
Dockerfile
FROM python:3.10
WORKDIR /app
COPY requirements.txt .
RUN pip install -r requirements.txt
COPY . .
CMD ["python", "app.py"]
💚 Go
Dockerfile:
FROM golang:1.20
WORKDIR /app
COPY . .
RUN go build -o main .
CMD ["./main"]
Simple, clean, and perfect for microservices.
Live Code Changes with Docker (Hot Reload)
You don’t want to rebuild your image every time you make a code change.
Use volume mounts to reflect your local changes instantly:
docker run -v $(pwd):/app -p 3000:3000 my-node-ap
Better yet — use nodemon
or similar tools inside your container for auto-reload.
Debugging Inside a Container
Need to poke around inside a running container?
docker exec -it bash
Or:
docker logs <container_id>
Pro tip: Always add logging early. You’ll thank yourself later.
CI/CD Pipelines With Docker
Docker fits naturally into CI/CD. You can:
- Build and push images in GitHub Actions
- Run tests inside containers
- Deploy via Docker Compose or Kubernetes
Example: GitHub Actions step
- name: Build Docker Image
run: docker build -t my-app:latest .
Then publish it to Docker Hub or GitHub Container Registry.
Why This Rocks for Teams
- Everyone works in the same environment
- Onboarding is instant
- Less “it works on my machine” drama
- Perfect setup for remote or hybrid teams
Section 6: When to Use Docker (vs Other Tools)
Docker is great but it’s not the only tool in the toolbox. Let’s break down when to use it, when to skip it, and what other options are out there.
TL;DR When You Should Use Docker
✅ You want consistent environments (dev = prod = CI)
✅ You’re running microservices or APIs
✅ You work with a team and want fast onboarding
✅ You want to avoid polluting your system with random installs
✅ You’re deploying to cloud providers or Kubernetes
When Docker Might Be Overkill
❌ You’re building a small static site or HTML page
❌ You just want to try out a quick Python script
❌ You don’t want to mess with configs and networks (right now)
❌ You’re on very limited RAM/older machine
❌ You’re already deep in Vagrant or a custom dev VM
Tool Comparison Table
https://miro.medium.com/v2/resize:fit:640/format:webp/1https://miro.medium.com/v2/resize:fit:720/format:webp/1*t86hOpagmhYwe5N9yNPlPQ.pnghttps://miro.medium.com/v2/resize:fit:750/format:webp/1*t86hOpagmhYwe5N9yNPlPQ.pnghttps://miro.medium.com/v2/resize:fit:786/format:webp/1*t86hOpagmhYwe5N9yNPlPQ.pnghttps://miro.medium.com/v2/resize:fit:828/format:webp/1*t86hOpagmhYwe5N9yNPlPQ.pnghttps://miro.medium.com/v2/resize:fit:1100/format:webp/1*t86hOpagmhYwe5N9yNPlPQ.pnghttps://miro.medium.com/v2/resize:fit:1400/format:webp/1*t86hOpagmhYwe5N9yNPlPQ.png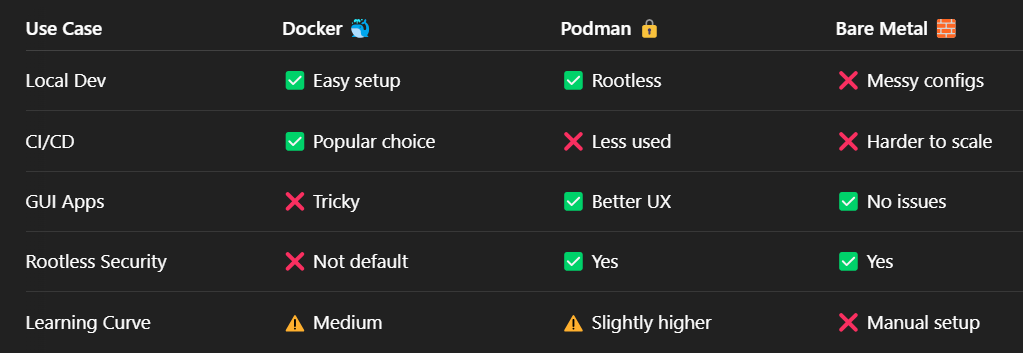
What About Docker Alternatives?
- Podman: Drop-in replacement for Docker, but runs rootless by default. More secure for paranoid devs or prod environments.
- Vagrant: Good for simulating full virtual machines (used to be more popular).
- Bare Metal: No containers. Everything is installed directly on your machine (why tho?).
Real Talk from Devs
“Docker saved us from the ‘works on my machine’ nightmare.” — every backend dev, ever
“If it takes more than 10 minutes to set up a dev environment, you need Docker.” someone on Stack Overflow probably
You don’t have to always use Docker but knowing when to reach for it makes you 10x more effective as a developer.
Section 7: Advanced Docker Tricks (Without Losing Your Mind)
By now, you’re running apps, spinning up services, and feeling like a container-wielding wizard. But there’s more under the hood. Let’s unlock the real power tools that make Docker great for production and big projects.
Multi-Stage Builds Slim Down Your Images
Ever build an image that’s 1.4GB because it included everything?
Multi-stage builds fix that.
Why use it?
Separate the build environment from the runtime — so you don’t ship unused tools or dev junk.
Example:
Dockerfile
# Stage 1: Build
FROM node:18 AS builder
WORKDIR /app
COPY . .
RUN npm install && npm run build
# Stage 2: Serve
FROM nginx:alpine
COPY --from=builder /app/dist /usr/share/nginx/html
Result?
✅ Fast
✅ Small
✅ Clean
This is especially useful in frontend builds and compiled languages (Go, Rust, etc.).
Docker Swarm Worth It?
Docker Swarm lets you scale containers across multiple machines — like baby Kubernetes.
But in 2025? It’s kinda faded out. Most teams now go straight to Kubernetes for orchestration.
If you’re working solo or want quick container scaling for demos, Swarm can still be handy.
Docker + Kubernetes
Docker is NOT Kubernetes, but it plays well with it.
What Docker gives you:
- Easy local dev
- Simple container builds
- Reproducible environments
What Kubernetes gives you:
- Powerful scaling & orchestration
- Self-healing apps (containers restart if they fail)
- Load balancing, secrets, config maps, and more
For serious deployment, most companies use:
- Docker → to build the images
- Kubernetes → to run and manage them
Try minikube or kind if you want Kubernetes locally.
Keep It Secure Don’t Get Owned
Docker is easy, but it also needs some care.
Security Tips:
- Avoid
:latest
tags – use versioned images (e.g.,nginx:1.25
) - Don’t run containers as root (use a user in your Dockerfile)
- Use tools like Trivy to scan your images
- Keep images slim less attack surface
- Don’t copy secrets into images
Speed Up Builds
- Use
.dockerignore
to skip unnecessary files - Put
COPY package.json
beforeCOPY . .
in Node projects - Cache dependencies in early layers
This can make your builds 10x faster.
Tools Every Advanced Docker User Loves
https://miro.medium.com/v2/resize:fit:640/format:webp/1https://miro.medium.com/v2/resize:fit:720/format:webp/1*njLvVbpnOeK-v9mbOPiSrQ.pnghttps://miro.medium.com/v2/resize:fit:750/format:webp/1*njLvVbpnOeK-v9mbOPiSrQ.pnghttps://miro.medium.com/v2/resize:fit:786/format:webp/1*njLvVbpnOeK-v9mbOPiSrQ.pnghttps://miro.medium.com/v2/resize:fit:828/format:webp/1*njLvVbpnOeK-v9mbOPiSrQ.pnghttps://miro.medium.com/v2/resize:fit:1100/format:webp/1*njLvVbpnOeK-v9mbOPiSrQ.pnghttps://miro.medium.com/v2/resize:fit:1400/format:webp/1*njLvVbpnOeK-v9mbOPiSrQ.png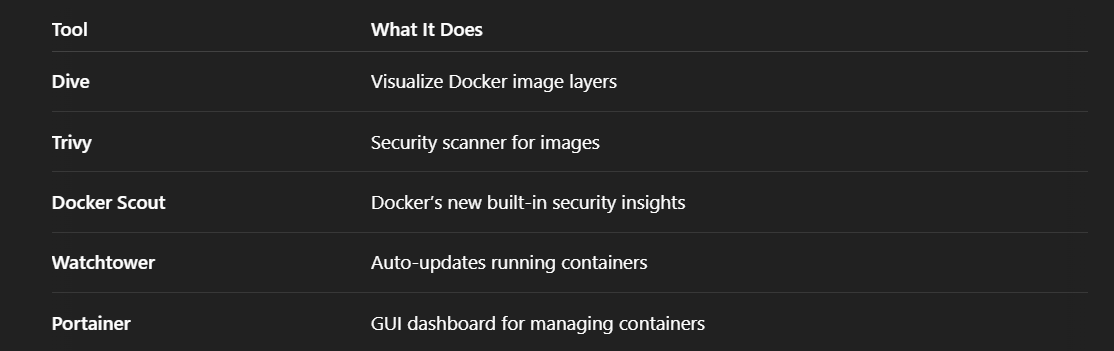
Section 8: Real Dev Use Cases (TL;DR Edition)
You’ve seen the features. You’ve learned the commands. But how does Docker actually fit into your day-to-day workflow?
Let’s walk through a few real dev scenarios where Docker saves time, avoids headaches, and makes you feel like a wizard.
1. 👨💻 Frontend Dev with Hot Reload (React/Vue)
The problem:
You’re working on a React app, but your teammate’s machine has a different Node version. And you’re tired of node_modules
drama.
The Docker solution:
- Create a containerized Node environment
- Mount your local code
- Use
nodemon
orvite
for hot reload
Dockerfile:
Dockerfile
FROM node:18
WORKDIR /app
COPY package.json ./
RUN npm install
COPY . .
CMD ["npm", "start"]
Run it live with volume:
docker run -v $(pwd):/app -p 3000:3000 my-react-app
No local Node version required. It just works™️.
2. Backend + Database Combo (Full-Stack)
Use case:
You’re building a backend (Node, Django, Flask) and need a DB like MongoDB or PostgreSQL.
Without Docker:
Install locally. Pray ports don’t conflict. Cry when switching projects.
With Docker Compose:
services:
backend:
build: .
ports:
- "5000:5000"
depends_on:
- db
db:
image: postgres
environment:
POSTGRES_PASSWORD: secret
One docker-compose up
and boom: your app and DB are up, linked, and working.
3. Local API + External UI
You’re building a Python API, and your designer is working on a Vue frontend. You want to test how they talk to each other.
Run your API in a Docker container. Share the IP/port with your teammate. Done.
Bonus: This mirrors production, so you catch CORS or network issues before deployment.
4. Hosting Your Own Tools (the fun stuff)
With Docker, you can spin up:
- ✅ PostgreSQL
- ✅ Redis
- ✅ Nginx reverse proxy
- ✅ Local Git server
- ✅ WordPress, Ghost, or even Minecraft
Example: Host a Ghost blog in one line:
docker run -d --name blog -p 2368:2368 ghost
Suddenly you’re self-hosting like a boss.
5. Shipping to Prod with Zero Pain
Build your Docker image locally, push to Docker Hub (or GitHub Container Registry), and deploy it anywhere.
No more:
“Wait, what version of Node is on prod?”
Now it’s:
“Here’s the image. It just runs.”
Section 9: Common Docker Mistakes Beginners Make (And How to Avoid Them)
Docker is awesome, but it’s easy to trip over the same issues again and again when starting out.
Here are the most common “oops” moments — and how to fix or avoid them.
1. Using :latest
for Everything
It seems smart… until your build breaks randomly one day.
Why it’s bad:
latest
is not a version — it just means "whatever version was built last." If the image updates silently, your code might break without warning.
✅ Fix:
Use fixed versions like node:18.17
or postgres:15
. It’s stable and predictable.
2. Forgetting to .dockerignore
Things
Ever build a Docker image and it takes forever?
You might be copying your entire .git
folder, node_modules
, and even logs/
.
✅ Fix:
Add a .dockerignore
file:
.git
node_modules
logs
.env
Your builds will be way faster and cleaner.
3. Running Everything as Root
It works, but it’s dangerous.
A misbehaving container could mess with your system if it’s running as root.
✅ Fix:
In your Dockerfile:
Dockerfile
RUN useradd -m appuser
USER appuser
4. Not Cleaning Up
Images and containers pile up like unwashed dishes.
✅ Fix:
Run these regularly:
docker system prune
docker image prune
docker volume prune
Or go full savage (⚠️ dangerous):
docker system prune -a --volumes
5. Confusing Image vs Container
You run an image, it fails. You fix your code. But the container still shows the old behavior.
Why? You didn’t rebuild the image.
✅ Fix:
After changing your code:
docker build -t my-app .
docker run my-app
Otherwise, you’re just rerunning the old stuff.
6. Hardcoding Secrets
Please. For the love of root access. Don’t do this:
Dockerfile
ENV DB_PASSWORD=mysecretpassword
✅ Fix:
Use Docker secrets (in Swarm or Kubernetes) or .env
files with Compose.
7. Too Much in One Container
Each container should do one thing well.
Not this:
docker run my-app-with-db-and-nginx-and-python-and-ftp
✅ Fix:
Split services using Docker Compose or Kubernetes.
Bonus Tip
Don’t panic. Everyone messes up early on — Docker just makes the mistakes faster (and easier to fix).
Section 10: Final Checklist, TL;DR & Resources
You made it. From running hello-world
to building production-ready images welcome to the Docker club 🎉
Before you sail off into the containerized sunset, here’s your cheat sheet and toolkit.
✅ TL;DR What You’ve Learned
- Docker lets you run apps in isolated, portable environments called containers.
- Images are the blueprint; containers are the running app.
- Use
Dockerfile
to build your own images. - Use volumes to save data, networks to connect containers, and Compose to manage multi-service apps.
- Docker works great for local dev, CI/CD, and cloud deployments.
- Avoid common mistakes: don’t use
:latest
, don’t forget.dockerignore
, don’t run everything as root. - Go advanced with multi-stage builds, Trivy for scanning, and Kubernetes if you’re ready to scale.
Final Checklist: Your Docker Skills Starter Pack
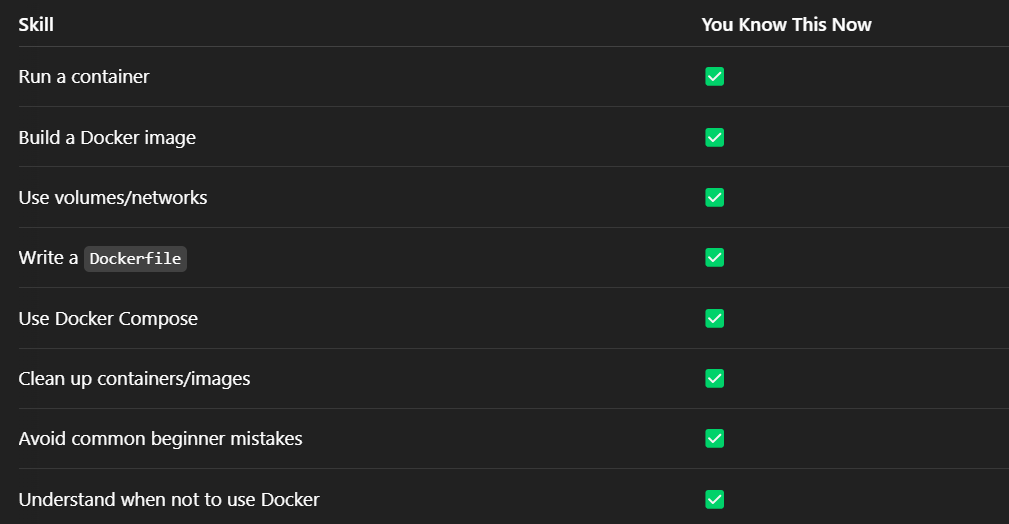
You’re officially more Docker-savvy than 90% of junior devs.
🔗 Helpful Resources to Bookmark
- Docker Official Docs: https://docs.docker.com/
- Play with Docker (sandbox): https://labs.play-with-docker.com/
- Dive (analyze image layers): https://github.com/wagoodman/dive
- Trivy (scan for vulnerabilities): https://github.com/aquasecurity/trivy
- FreeCodeCamp Full Docker Tutorial: YouTube Link
- Docker Cheat Sheet: https://github.com/wsargent/docker-cheat-sheet
Final Thoughts
Docker isn’t just for DevOps pros it’s for every developer who wants their setup to just work. Once you get the hang of it, you’ll never want to go back to messy local environments again.
Keep experimenting. Break stuff. Rebuild. That’s how you get good.
And next time someone on your team says:
“It works on my machine.”
You’ll smile, spin up a Docker container, and say:
“It works on mine too.”
Enjoyed this story?
If you liked it, please leave a comment sharing what topic you’d like to see next!
Feel free to like, share it with your friends, and subscribe to get updates when new posts go live. 🚀